TensorFlow 是用於機器學習的端對端開放原始碼平台
無論你是新手還是專家,TensorFlow 都能讓你輕鬆建立機器學習模型。如要開始使用,請參閱下列各節。
適合新手
The best place to start is with the user-friendly Sequential API. You can create models by plugging together building blocks. Run the “Hello World” example below, then visit the tutorials to learn more.
To learn ML, check out our education page. Begin with curated curriculums to improve your skills in foundational ML areas.
import tensorflow as tf mnist = tf.keras.datasets.mnist (x_train, y_train),(x_test, y_test) = mnist.load_data() x_train, x_test = x_train / 255.0, x_test / 255.0 model = tf.keras.models.Sequential([ tf.keras.layers.Flatten(input_shape=(28, 28)), tf.keras.layers.Dense(128, activation='relu'), tf.keras.layers.Dropout(0.2), tf.keras.layers.Dense(10, activation='softmax') ]) model.compile(optimizer='adam', loss='sparse_categorical_crossentropy', metrics=['accuracy']) model.fit(x_train, y_train, epochs=5) model.evaluate(x_test, y_test)
適合專家
The Subclassing API provides a define-by-run interface for advanced research. Create a class for your model, then write the forward pass imperatively. Easily author custom layers, activations, and training loops. Run the “Hello World” example below, then visit the tutorials to learn more.
class MyModel(tf.keras.Model): def __init__(self): super(MyModel, self).__init__() self.conv1 = Conv2D(32, 3, activation='relu') self.flatten = Flatten() self.d1 = Dense(128, activation='relu') self.d2 = Dense(10, activation='softmax') def call(self, x): x = self.conv1(x) x = self.flatten(x) x = self.d1(x) return self.d2(x) model = MyModel() with tf.GradientTape() as tape: logits = model(images) loss_value = loss(logits, labels) grads = tape.gradient(loss_value, model.trainable_variables) optimizer.apply_gradients(zip(grads, model.trainable_variables))
常見問題的解決方案
探索可協助你完成專案的逐步教學課程。
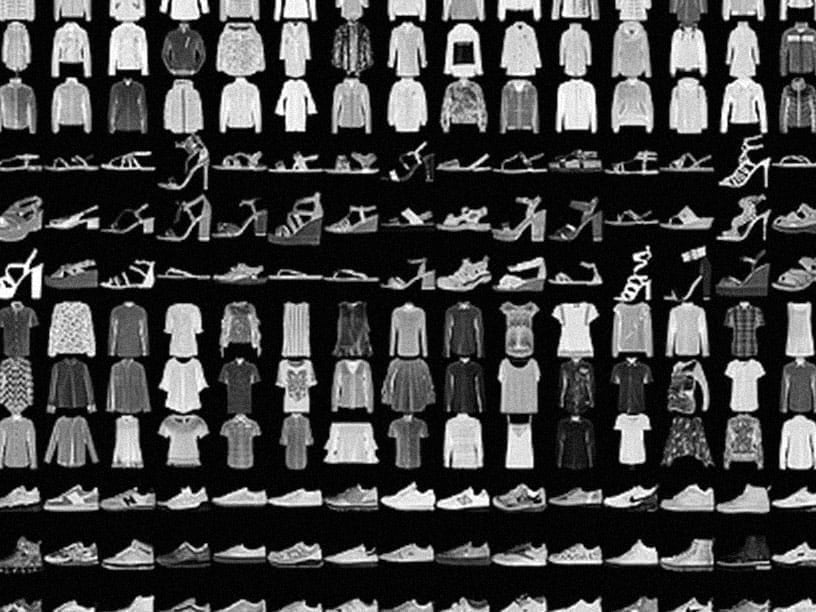

Generate images based on a text prompt using the KerasCV implementation of stability.ai's Stable Diffusion model.

Preprocess WAV files and train a basic automatic speech recognition model.